I recently built a site that let me explore the relatively new WordPress features of post thumbnails, along with the ability to auto scale/crop images while they are getting uploaded to WordPress.
The site, ImageAsheville, called for an unusual design, one similar to the fantastic AutoFocus WordPress theme (which I used as a basis for this alteration), but instead of doing very complicated functions.php code to find the first image in a post, I wanted to use the new featured image capabilities in WordPress.
The challenge to get the “autofocus effect” is that each entry in the loop needs to pull the featured image, but at a different size. The newest entry has a wide image, while as the posts get older we use smaller and smaller images. There are actually 6 image sizes that need to be generated when uploading a new featured image.
Step 1: Enable the Post Thumbnails in your function.php in your theme.
Adding support to your theme is quite easy, just add the following line to your functions.php.
[php]
add_theme_support( ‘post-thumbnails’ );
[/php]
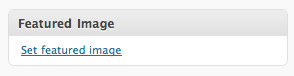
By enabling this part of wordpress, you will suddenly have a new admin sidebar available when you edit a post or page, the Featured Image sidebar. (below the publish, tags and category tools normally in wordpress).
Clicking the sidebar lets you choose an image and make it the “Featured Image” for your page or post. You can reference in your theme the post’s featured image with a set of theme functions. An example is showing the Featured Image in your category loop or on your homepage blog loop.
Full documentation on this can be found in the WP codex
Step 2: Add in the default image size for your featured image.
WordPress has some standard sizes for images (you have probably seen these when you upload an image and import them into WP, you have the thumbnail size, small, medium, etc…)
[php]
set_post_thumbnail_size( 100, 100 );
[/php]
We can use this to manually set the size of the post thumbnail default size this way. In the example above, it will scale your thumbnails while keeping the aspect ratio (the longest side will be 100px, the other will be whatever it has to be to keep the same aspect ratio).
By adding a true as the third parameter:
[php]
set_post_thumbnail_size( 100, 100, true );
[/php]
the images will be cropped exactly to the size indicated. This is what I wanted for the ImageAsheville site. The div’s on the frontpage are exact in size, and I like the artistic effect you get when you crop an image automatically.
Step 3: Add any other sizes you need in various templates.
So, now that your default thumbnail size is correct, you’ll want other sizes of images for various templates. These are added once again in your functions.php file, using the add_image_size() WP function. Here’s the entries for ImageAsheville:
add_image_size( ‘p1’, 600, 300, true );
add_image_size( ‘p2’, 419, 300, true );
add_image_size( ‘p3’, 374, 300, true );
add_image_size( ‘p4’, 334, 300, true );
add_image_size( ‘p5’, 306, 300, true );
add_image_size( ‘p6-9’, 251, 300, true );
[/php]
Step 4: Add the new images sizes to your template.
So, now that we have all the sizes we actually need for our templates, we can then add them to our template files. Use the the_post_thumbnail( ) function to call the default thumbnail inside your loop. You can also call any particular size you need with the same function by passing the name to the_post_thumbnail() like this:
[php]
<?php the_post_thumbnail(‘p1’); ?>
[/php]
That would, in our example, link to the the 600x300px image we created above (called p1).
Advanced: Extra coding for ImageAsheville
On ImageAsheville, each slot in our frontpage is generated, just like normal wordpress, from calling the loop. I needed to loop though all the newest articles, and create a counter so I would know what size image to put in the section of the page. Also, the last row of images (6-9) are all the same size slots, so I set count to the string “6-9” if we are that far.
[php]
<?php
$i++;
if ($i >= 6){ $count = "6-9"; }
else { $count = $i; }
?>
[/php]
Then, using the counter to call the correct thumbnail image at the right size: [php]
<span class="attach-post-image p<?=$count?>">
<?php the_post_thumbnail( ‘p’.$count ); ?>
</span>
[/php]